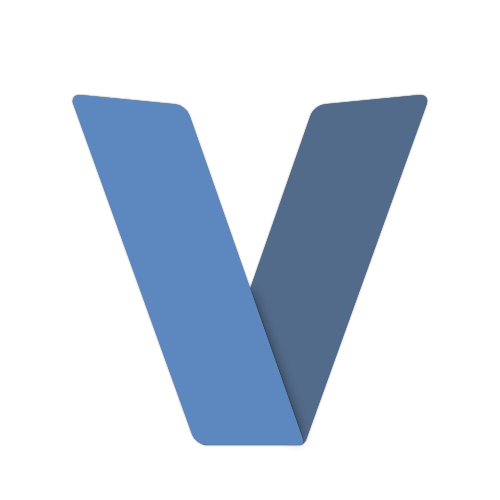
One of my chief gripes about modern development is the disk and memory bloat that so often seems to come with modern tool chains. I like having access to cross platform managed languages like Kotlin and C#. I appreciate but don’t love the language offerings for doing the same things with JavaScript and TypeScript. These languages though are positively bloated compared to old fashioned C and C++. Is that just the price of doing business? I used to think so until recently. A few weeks ago OSNews posted this story on a new OS called Vinix which is trying to write a whole OS in a language called V , in the same way that Redox is trying to do it with Rust. I never heard of the language but became intrigued with an initial look. The more I read about it and begin experiments with it the more I like.
This is always an on again off again fad for me. I spent a lot of my early career writing C/C++ code. Yes by being careful and such you can avoid stubbing your toe with memory leaks, walking off end of arrays, etc. but it isn’t that hard to be careful and still screw up. Yes C/C++, especially C, is highly portable across many platforms, but it comes at the cost of having unmanaged code. I therefore have considered that Rust, Go, or Kotlin Native would probably be the only way for me to get something akin to native C development but with the benefits of a higher level language. They all have their pluses and minuses. V is just one more added to the list. One thing I like about V is that it is very small and self contained. Builds are currently fast and it is a very thin layer on top of smaller compiler chains. The executables themselves are incredibly small too. Executable disk footprints are measured in KB not MB. Memory footprints are too. The real appeal to me though is that it compiles down to human readable C code.
It’s not pretty human readable C code but it is understandable C code. This allows one to hypothetically bring the C code intermediary over to a computer that doesn’t have your V build environment and run there as long as it has an ANSI C compiler. The code will be self contained with the convenience libraries etc bundlede in entirely. That sounds very bloated but in my initial trials is still much smaller than code generated from the other languages. The syntax feels very Go/Rust/Kotlin like. It doesn’t do garbage collection but does hide the raw C style data accesses to prevent one from falling into the common toe stubbing problems. That really is the beauty of it. I can get things like string interpolation, map/reduce operations on collections, standardized collections for arrays/maps/sets, all in a small cross platform way. It even provides some semblence of object oriented programming or interface oriented programming by allowing one to add methods to structs (which can’t be subclassed) and interfaces. You get a hint of that in the code sample on their website:
fn main() {
areas := ['game', 'web', 'tools', 'science', 'systems',
'embedded', 'drivers', 'GUI', 'mobile']
for area in areas {
println('Hello, $area developers!')
}
}
In there you see a foreach statement. You see the string interpolation. You see the array in place creation. You see much more modern syntax. But this does compile down to straight C that is human readable. Here is this function in the generated C file:
VV_LOCAL_SYMBOL void main__main(void) {
Array_string areas = new_array_from_c_array(9, 9, sizeof(string), _MOV((string[9]){
_SLIT("game"), _SLIT("web"), _SLIT("tools"), _SLIT("science"), _SLIT("systems"), _SLIT("embedded"), _SLIT("drivers"), _SLIT("GUI"), _SLIT("mobile")}));
// FOR IN array
for (int _t732 = 0; _t732 < areas.len; ++_t732) {
string area = ((string*)areas.data)[_t732];
println( str_intp(2, _MOV((StrIntpData[]){{_SLIT("Hello, "), 0xfe10, {.d_s = area}}, {_SLIT(" developers!"), 0, { .d_c = 0 }}})) );
}
}
It isn’t elegant from a human generated perspective but it is definitely readable. It is also compilable by just doing gcc main.c
which means it is self contained. Granted these ten lines are “self contained” in a 358 KB C file with just over 10,000 lines. That is still more transportable, hypothetically anyway, than the alternatives. Along with the core language with lots of library functions for doing things like JSON parsing, ORM, REST calls, encryption (calling into native OS C libraries), it also has functionality for concurrency akin to Go’s, easy calling of C code (because it is just making C code), and some other niceities like package management. VPM
is the package manager built into the V compiler and the cloud based service for hosting those repositories. There are libraries for making cross platform UIs V-UI
to interacting with Objective C. Hypothetically you can make cross platform applications not just for desktops and servers but also for iOS and Android mobile devices.
While V has a lot of potential the developers will be the first to tell you it is still in a relatively raw state and under heavy development. There are language plugins for VS Code but they aren’t as full featured as one may expect from other languages. There is no native debugger system that works with V in lingua franca but there are ways around that due to it’s C-code generation and some other language features that can help with “printf style” debug logging. Things like V-UI and other libraries are also in an alpha state. There are some larger under the covers changes for things like the memory management handling even though the language syntax itself is pretty set in stone, as they say here . I’ve found the community on Discord to be very friendly and helpful when I was dabbling with things and to help get past some of this rawness but the rawness is still just a fact of life at its current state of maturity.
Next Steps
The Windows 11 release has me thinking a lot about bloat again. The new OS has a bare minimum requirement of 2 cores, 4 GB of RAM, and 64 GB of HD space. We know that minimum requirements are usually barely sufficient. Why is it so bloated? Likewise I look at some of these jar files I’m building nowadays. My simple Git Stat generator isn’t too bad but still a bit bloated at 4.6 MB for a self conatined JAR file. That of course needs a runtime on the computer that is executing it. Although V is too early for production it’s not too early for me to want to experiment with it and contribute to it. Things I want to do in the very short term include:
- Carefully do their web service/site tutorial to fix small errors I’ve discovered while executing it
- Write some sample code in Kotlin and V to get performance and size metrics
- Test portability by trying to compile/run programs on various legacy platforms or esoteric platforms: OPENSTEP, FreeDOS, Haiku, SerenityOS
- Write a Twitter interactive tutorial and blog about the details
- Port my Facebook Partisanship Calculator from Kotlin to V to test real world capabilities
- Help with any bugs/feature requests I find along the way with the above